직렬화(Serialization) : 객체를 파일에 저장하려면 객체가 가진 data들을 순차적인 data로 변환하는 과정
역직렬화(Deserialization) : 직렬화된 data를 읽어서 자신의 상태를 복구하는 것
변수의 초기화
멤버변수는 초기화를 하지 않아도 자동적으로 변수의 자료형에 맞는 기본값으로 초기화가 이루어지므로 초기화하지 않고 사용해도 되지만 지역 변수는 사용하기 전에 반드시 초기화를 해야한다.
class InitTest {
int x;
int y = x; //문제 없음
void method() {
int i;
int j = i; // 컴파일 에러: 지역변수를 초기화하지 않고 사용함
}
}
String str1 = "asdf";
String str2 = "asdf";
String str3 = new String("a");
String str4 = new String("a");
System.out.println(str1==str2); //true
System.out.println(str1.equals(str2)); //true
System.out.println(str3==str4); //false
System.out.println(str3.equals(str4)); //true
Q. 하나의 파일에서 public class는 1개 밖에 못하는데 public이 가지는 뜻이 뭘까?
Q.java.io.NotSerializableException
네트워크 상에서 동작하는 것들은 java.io.Serializable 상속 받아라 //객체읽고 쓰기 위해서 직렬화 가능
Q. 객체를 print하려고 하는데 분명 sysout을 했는데 toString()을 왜 Override 해줘야할까?
=> So, long story short, System.out.println(someObject) calls that object's toString() function to convert the object to a string representation.
==> sysout은 toString()을 요청하기 때문이다.
Q. toString, toArray 이런거 쓸 때 객체 앞에 강제 형변환 시켜주는데 이런거 왜함?
별도의 오버라이딩을 하지않으면 Object의 메소드인데 자식 클래스인 애들이 부모클래스 메소드를 오버라이딩 안하고 쓰게 되면 문제가 생기기 때문에(cannot convert from Object[] to Book[]) 이런식으로 해야됨
return (Book[])books.toArray();
내가 막 특정한 자료형을 만들어서 활용하고 싶을 때 어떻게 활용하면 될까?
class Pair {
private int x;
private int y;
Pair(int x, int y) {
this.x = x;
this.y = y;
}
public int getX(){
return x;
}
public int getY(){
return y;
}
}
<Input>
Node : 입력과 출력의 끝단
Stream : 두 노드를 연결하고 데이터를 전송할 수 있는 개념
Node stream : node에 연결되는 스트림
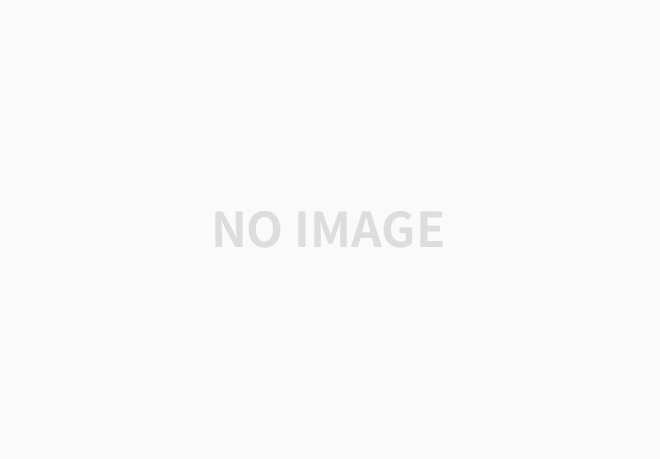
1. ConsoleRead - ConsoleWrite
package io;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
public class ConsoleReadConsolWrite {
//node stream(System.in : InputStream) -> filter stream(InputStreamReader) -> filter stream(BufferedReader) -> readLine() -> close
//node stream(System.out : PrintStream) -> filter stream(OutputStreamReader) -> filter stream(PrintWriter) -> println -> close
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out),true);
String data = null;
try {
while ((data = br.readLine()) != null) { // EOF
// System.out.println(data);
out.println(data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (br != null)
br.close();
} catch (IOException e) {
e.printStackTrace();
}
if (out != null)
out.close();
}
}
}
2. ConsoleRead - FileWrite
package io;
import java.io.BufferedReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintWriter;
public class ConsoleReadFileWrite {
//node stream(System.in : InputStream) -> filter stream(InputStreamReader) -> filter stream(BufferedReader) -> readLine() -> close
//node stream(File : FileWriter) -> filter stream(PrintWriter(out:Writer, autoflush:boolean)) -> println() ->close
public static void main(String[] args) {
BufferedReader br = null;
PrintWriter out = null;
try {
br = new BufferedReader(new InputStreamReader(System.in));
out = new PrintWriter(new FileWriter("consoleInput.txt"), true);
String data = null;
//String data; 로 써도 오류 안나던데 null로 알아서 초기화 되는거 아닌가?
while ((data = br.readLine()) != null)
out.println(data);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (br != null)
br.close();
if (out != null)
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
3. FileRead - FileWrite(FileCopy)
package io;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
public class FileCopy {
//node stream(FileReader) -> filter stream(BufferedReader) -> readLine() -> close()
//node stream(FileWriter) -> filter straem(PrintWriter) -> println() -> close()
public static void main(String[] args) {
BufferedReader br = null;
PrintWriter out = null;
String data = null;
try {
br = new BufferedReader(new FileReader("consoleInput.txt"));
out = new PrintWriter(new FileWriter("copy_console.txt"));
while ((data = br.readLine()) != null)
out.println(data);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (br != null)
br.close();
if (out != null)
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
래퍼 클래스(Wrapper class)
프로그램에 따라 기본 타입의 데이터를 객체로 취급해야 하는 경우가 있음
8개의 기본 타입에 해당하는 데이터를 객체로 포장해 주는 클래스를 래퍼 클래스(Wrapper class)
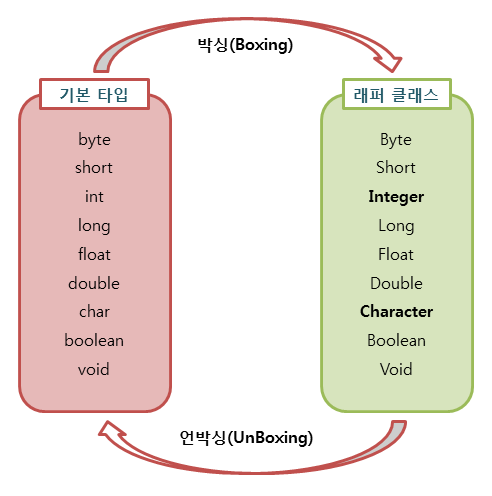
오토 박싱(AutoBoxing)과 오토 언박싱(AutoUnBoxing)
JDK 1.5부터는 박싱과 언박싱이 필요한 상황에서 자바 컴파일러가 이를 자동으로 처리하며
이렇게 자동화된 박싱과 언박싱을 오토 박싱(AutoBoxing)과 오토 언박싱(AutoUnBoxing)이라고 부른다
오토 박싱을 이용하면 new 키워드를 사용하지 않고도, 반대로 intValue() 메소드를 사용하지 않고도 박싱/언박싱이 가능
Integer num = new Integer(17); // 박싱
int n = num.intValue(); // 언박싱
System.out.println(n);
Character ch = 'X'; // Character ch = new Character('X'); : 오토박싱
char c = ch; // char c = ch.charValue(); : 오토언박싱
System.out.println(c);
== 연산자는 안됨. .equal() 써야됨(클래스이기 때문에)
Wrapper Class 왜 씀?
// 1. Generic에서 우린 쓰고있음(오토언박싱 때문에 바로 int로 쓸수있던거임)
ArrayList<Integer> arrayList = new ArrayList<Integer>();
arrayList.add(10);
arrayList.add(new Integer(100));
Integer it = arrayList.get(1);
int it2 = arrayList.get(1);
System.out.println(it);
System.out.println(it2);
// 2. 메소드 편하게 사용하려고
int i = 12;
String str1 = Integer.toString(i);
Integer j = 12;
String str2 = j.toString();
<Enum class>
상수 List라고 보면 되는데 쉽게 말해서 자신이 정한 상수 List라고 보면됨
ex) 쇼핑몰에서 등급을 정할 때 'Gold', 'Sliver' ... 이런게 있는데 하나하나의 값들은 String이지만 {'Gold', 'Sliver' ...}처럼 바뀌지않는 상수를 모아둔 자료구조를 Enum이라고함
enum Rainbow { RED, ORANGE, YELLOW, GREEN, BLUE, INDIGO, VIOLET }
Rainbow[] arr = Rainbow.values();
for (Rainbow rb : arr) {
System.out.println(rb);
}
<String Class>
charAt()
charAt() 메소드는 해당 문자열의 특정 인덱스에 해당하는 문자를 반환
//In python에서 list[i]로 접근하는 것을 str.charAt(i)로 하는것임
String str = new String("Java");
for (int i = 0; i < str.length(); i++)
System.out.print(str.charAt(i) + " ");
compareTo()
compareTo() 메소드는 해당 문자열을 인수로 전달된 문자열과 사전 편찬 순으로 비교한다
String str = new String("abcd");
System.out.println(str.compareTo("bcef")); // 음수
System.out.println(str.compareTo("abcd")); // 0
System.out.println(str.compareTo("Abcd")); // 양수
//대소문자 무시하여 compareTo
System.out.println(str.compareToIgnoreCase("Abcd")); // 0
toCharArray() - String을 배열에 바로 저장
string data = "abcd";
char data[] = data.toCharArray();
equals()
객체의 주소가 아닌 내용을 가지고 값이 같은지 검사를 하는 것
Q. 객체에 여러가지 변수가 있을 때 equals을 쓰면 어떻게 될까?
보통 문제를 풀면 string하나씩만 비교하는게 아니라 어디 리스트나 튜플에 넣어서 그 값들 전체가 같냐 다르냐 하는데 이걸 for돌려서 각각 값에 대해 참조를 해야할까?
그냥 Type[] 같이 따로 선언하지 않고 사용하는 배열같은 참조형 변수들안에 있는 값들을 비교하려고 하면 for문을 돌려서 비교하는게 정석이지만 다른 방법이 있는지 탐구 하는 목적임
==> 내가 다양한 자료형을 쓰려고 따로 만든 Test라는 Class에 대해서는 equals를 Override해서 써야한다.
why?)
//Object Class
public boolean equals(Object obj){
return (this == obj);
}
Q. 그냥 equals()을 쓰게 되면 Object의 equals()을 가져와서 주소값을 비교하는건데 왜그럴까?
String끼리 equals()하면 주소값비교인데 왜 같다고 나오지? ==> String Class안에 equals()을 오버라이딩 해놨기 때문
즉, 우리가 만든 Pair Class도 오버라이딩 해줘야됨
indexOf()
indexOf() 메소드는 해당 문자열에서 특정 문자나 문자열이 처음으로 등장하는 위치의 인덱스를 반환
만약 해당 문자열에 전달된 문자나 문자열이 포함되어 있지 않으면 -1을 반환
//list.index(i)
String str = new String("Oracle Java");
System.out.println("원본 문자열 : " + str);
System.out.println(str.indexOf('o')); //-1
System.out.println(str.indexOf('a')); //2
System.out.println(str.indexOf("Java")); //7
trim()
trim() 메소드는 해당 문자열의 맨 앞과 맨 뒤에 포함된 모든 공백 문자를 제거
toLowerCase() && toUpperCase()
<Math Class>
Math.max() Math.min()
System.out.println(Math.max(3.14, 3.14159)); // 3.14159
System.out.println(Math.min(3.14, 3.14159)); // 3.14
Math.pow() Math.sqrt()
System.out.println((int)Math.pow(5, 2)); // 25
System.out.println((int)Math.sqrt(25)); // 5
Math.random()
random() 메소드는 0.0 이상 1.0 미만의 범위에서 임의의 double형 값을 하나 생성하여 반환
System.out.println((int)(Math.random() * 100)); // 0 ~ 99
//Math class말고 Random Class 자체를 사용 가능
Random ran = new Random();
System.out.println(ran.nextInt(100)); // 0 ~ 99
Math.abs()
System.out.println(Math.abs(10)); // 10
System.out.println(Math.abs(-10)); // 10
System.out.println(Math.abs(-3.14)); // 3.14
Math.ceil() Math.floor() Math.round()
올림 내림 반올림
//올림
System.out.println(Math.ceil(10.0)); // 10.0
System.out.println(Math.ceil(10.1)); // 11.0
System.out.println(Math.ceil(10.000001)); // 11.0
//내림
System.out.println(Math.floor(10.0)); // 10.0
System.out.println(Math.floor(10.9)); // 10.0
//반올림
System.out.println(Math.round(10.0)); // 10
System.out.println(Math.round(10.4)); // 10
System.out.println(Math.round(10.5)); // 11
<Arrays Class>
Arrays.binarySearch()
전달받은 배열에서 특정 객체의 위치를 이진 검색 알고리즘을 사용하여 검색한 후, 해당 위치를 반환
당연히 이진 탐색 알고리즘이기 때문에 sort() 되어있는 상태에서 해야만 제대로 동작하게 된다.
System.out.println(Arrays.binarySearch(arr, 437));
Arrays배열 특정 범위 자르기()
- 배열에서 특정 범위를 자르고, 다른 배열에 저장할 때 Arrays.copyOfRange(배열명,시작점,끝점) 메소드를 사용한다.
- 이때, 범위는 [시작점,끝점) 형식으로 시작점 이상 끝점 미만의 범위가 설정된다.
int[] array={1,2,3,4,5};
int[] temp=Arrays.copyOfRange(array,1,3);
System.out.println(temp);
[출력]
[2,3]
Arrays.deepEquals(arr1,arr2)
2차원 배열 값 모두 맞는지 비교
2차원 배열 row단위로 초기화 할 때 3번처럼 x
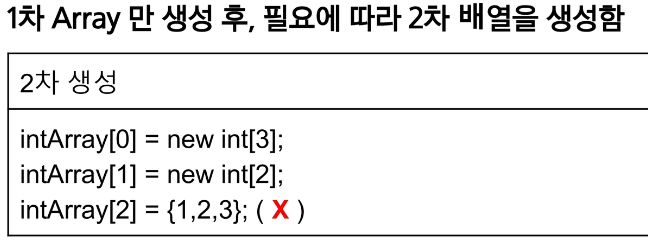
형 변환
● Java int to x
//Java int to String
Integer.toString(123)
//Java int to char,long,float,double ...
(char),(long),(float) ...
● Java char to x
//Java char to int
Character.getNumericValue('5') //메소드 접근
'5' - '0' //아스키 코드
// Java char to String
Character.toString('5')
● Java string to x
//Java string to int
Integer.parseInt("123");
//Java string to float
Float.parseFloat("123");
//Java string to char
string data = "1234";
char c = data.CharAt(index);
//valueOf
Integer x =Integer.valueOf(9); //9
Double c = Double.valueOf(5); //5.0
Float a = Float.valueOf("80"); //80.0
Integer b = Integer.valueOf("444",16); //1092
● Arrays.toString() - 2차원 배열 원소 출력
Arrays.toString(arr)
[[I@762efe5d, [I@5d22bbb7, [I@41a4555e]
Arrays.deepToString(arr)
[[2, 3, 1, 4, 7], [8, 13, 3, 33, 1], [7, 4, 5, 80, 12], [17, 9, 11, 5, 4], [4, 5, 91, 27, 7]]
//3차원 이상은?
● Deep copy : Arrays.copyOf(), System.arraycopy
scores = Arrays.copyOf(arr, 5);
System.arraycopy(scores, 0, scores2, 0, scores.length);
불필요한 import 나 변수 선언을 없애는 단축키
ctrl + shift + o
들여쓰기 정렬
ctrl + shift + f
객체를 비교할 때 ==로 비교하게 되면 Hash code값을 비교함
값을 비교할때는 Object.equals()로 해야됨
ctrl shift / : 주석
ctrl shift \ : 주석해제
추상클래스는 자신의 클래스로 생성자 할 수 없음
'Backend > Java' 카테고리의 다른 글
Java 직렬화 (0) | 2022.07.27 |
---|---|
Java 예외 처리 (0) | 2022.07.26 |
Java Generic, Collection에 대해 (0) | 2022.07.26 |
Java Override & Overload (0) | 2022.07.20 |
JDK란? JRE, JVM에 대한 정리 (0) | 2022.07.16 |